Android :: Access Values Defined In A Java Manifest From Code?
Feb 4, 2010Can I access the values defined in a java manifest from code?
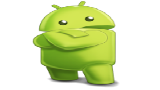
Can I access the values defined in a java manifest from code?
I defined a white color in mycolors.xml under res/values as below:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="my_white">#ffffffff</color>
</resources>
In my code, I set the text color of a TextView as the white color I defined:
TextView myText = (TextView) findViewById(R.id.my_text);
myText.setTextColor(R.color.my_white);
But the text in the TextView turned out to be a black color. When I use @color/my_white in layout xml files, it works fine. I check the generate R.java, and see: public static final int my_white=0x7f070025;
I'm developing an Android application that I'd like to be compatible with 1.5 (SDK version 4).
I'm testing the application on 2.2 (SDK version 8).
To do this, I'm including in the manifest file the line <uses-sdk android:minSdkVersion="4" android:targetSdkVersion="8" />
I thought this would allow me to use the newest manifest elements and APIs, but I'm getting a compile error whenever I try to use them. For example, I try to define the element installLocation to allow the app to be installed on the SD card, but Eclipse gives me the error
No resource identifier found for attribute 'installLocation' in package 'android'
Is there something else I have to do to get this to work? If I can't get this to work, what benefit is defining targetSdkVersion?
Is it possible to define a ListPreference in Xml and retrieve the value from SharedPreferences using getInt? Here is my Xml code...
And I want to get the value with something like: int val = sharedPrefs.getInt(key, defaultValue).
At the moment I have to use getString and parse the result.
I want to access the build type [release/debug] in java code and xml file to enable or disable the particular feature at the run time.
Please let me know if we have any environment variable defined for it which can be accessed in java code and in xml file also.
I am writing a adapter for a specific app, the problem I met is:
I have only 1 listview in main.xml, and the row style is defined in file_row.xml. If I simply use list.setAdapter(new ArrayAdapter<String>(this, R.layout.file_row, R.id.file_name, items)); where items is a ArrayList<String>, everything works well.
But if I use my custom Adapter, say, list.setAdapter(new MyOwnListAdapter(this, filename)); and in the class of MyOwnListAdapter, the code has been simplified as
CODE:................
But I always met the problem which is:
CODE:.........
I thought the self-defined Adapter class actually has only one line of valid execution, which is super(context, R.layout.file_row, R.id.file_name, filename_list); It's essentially identical to direct calling of list.setAdapter(new ArrayAdapter<String>(this, R.layout.file_row, R.id.file_name, items)); Then why I always got this error if I call my own Adapter?
How can i request the versionName stored in manifext.xml from within my Code?
>manifest.xml
The AndroidManifest.xml contains the version name of the application, something like: android:versionName="1.0"
Now the question - is it somehow possible to access this version name in the source code, so that I can display it for example in an About Dialogue?
Is it possible to pass user defined Intend actions?
That is instead of using predefined Intend actions like "ACTION_MAIN" can I create my own action and use it in my code?
If so how do I do that?
I have been surfing for this detail for long but dint find anything useful. Can any of u help me regarding this?
I have a app that uses ads. The ad id is set in the manifest id with a meta-data tag. I want to change this tag when i start the app in some cases (diferent id for some localisations) Can i do this in code?
View 1 Replies View RelatedHow do you retrieve the current version code of an app's manifest? I don't need to access another application, I'm talking about My app accessing its own version code.
View 5 Replies View RelatedI would like to block either:
The entire cellphone carrier Sprint The specific phones Sprint Hero and Sprint Moment
Is there a way to do this in the manifest file or any other code related means?
I want to block the Sprint Hero for the widget bug and the Moment for handling contacts incorrectly. A huge fail on the part of Sprint.
Is there a right associated with write/read access to the sd card that needs to be set in the manifest.xml file? I'm currently trying to write to the sd card using a standard FileOutputStream here:
FileOutputStream out = new FileOutputStream("/ sdcard/images/"+imageName);
bmp.compress(Bitmap.CompressFormat.JPEG, 90, out);
But it doesn't seem to be working. It just says the location isn't found, which can happen if there are no permissions to read/write to the location.
implicit intent with class name as action defined in intentfilter. is not working. Only it it is defined as "android.intent.action." it is picked up ? is it so ?
I have app A with the following in manifest.xml file
CODE:.............
In app B, i tried to call the activity in A using below code.
CODE:.............
This code works. But not above line.
Does anybody know of a work around for the android xml file string array inability to process string <item>'s that are not legal java variable names If not, is this not a major bug? Why does an item in an array need to generate a reference id anyway? Isn't this redundant?
View 2 Replies View RelatedI want to refer to a static Java variable in my styles.xml, is that possible? Here's a pseudo-xml example:
<style name="Artwork">
<item name="android:background">@drawable/border_{Constants.MY_COLOR}</item>
</style>
Is it possible to change the minWidth/minHeight values in Java context (not with XML widget definition)?
View 2 Replies View RelatedI have read about using a 'dip' scale rather than just px, this is straightforward to implement in XML. But how do I adjust values accordingly in the java code? For example:
tv.setTextSize(20)
will result in text of size 20 px right? What's the quickest way of making this 20 dip, other than adding a resource?
I recently upgraded my Android app to support multiple resolutions. Previously, my Android.manifest file had a line:
To support multiple density and resolution devices, I changed this to:
CODE:............
I then added a couple of new directories, like drawable-hdpi-v4 and drawable-long-hdpi-v4 that includes the high-res versions of the graphics. That's about it.
Ever since releasing this update, there have been a decent number of users complaining about various problems:
the app icon doesn't appear (I did not create a high res version of the icon)
the home screen widget no longer works, even if they delete and re-add it (this code did not change with the update). I've had a user send me their error log, which shows:
CODE:................
There is one questionable section in my existing widget code that may be relevant:
CODE:..........
And perhaps most troublesome: the sqlite database is no longer accessible/writeable for some users so their data is no longer available. I did add the WRITE_EXTERNAL_STORAGE permission to the manifest. This is only happening to certain users and it tends to be HTC Eris users. In that error log I see things such as:
CODE:.........
It's as if the update has caused a new process and it can't access the old process's data, or something.
In my Android app, a Service runs in the background and logs GPS readings to a database. The user sees an Activity that presents them with radio buttons. I'd like to also log their currently selected radio button to the database. How can I access the radio button object from the Service?
View 1 Replies View RelatedI'm kinda noob to android so please bear with me. I'm currently developing app which uses tabs. My question now is: how to store values of variables so I can access them on the other tabs? I want to create something similar to sessions in PHP where I can save variables on one page and access on the other.
View 4 Replies View RelatedI don't know where to put the bug report of APIs.
I use WebView to display some pages in my dictionary software. I met some problems with WebView.
1) could not handle the color values When I use the HTML code: <font color="#00FF00"></font> the WebView could not handle this type of tags. But <font color="red"></font> can work well.
This bug exist both in SDK1.0 & SDK1.1
2) Chinese Char: I use UTF-8 as the charset, and if there exist some Chinese chars, then this HTML code could not be displayed in WebView: <html><head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"></head> <body> <p><font color="red">[-------- 美国 统词典[双解] --------]</font></p> </body> </html>
This bug exists in SDK1.1.
I can access android.R.string.ok value from java code, but can't see how to do the same in layout xml file - I only have @string/xxx in autocompletion list which does not have system string values.
View 3 Replies View RelatedI'm currently learning android and java, and I am having some issues with importing a java file.
I am working with the time and date example in the android api demos, and I want to put all the code relating to creating the time and date dialogs setting the values etc into a separate java file, and in my main java file run when the application starts, just declare the buttons and onclick events.
What is the best way to do this? Do I need to put all the code inside a class(that extends activity) or method? How do I use this inside my main class. Code...
Does anyone know how to access programatically the internal(flash) and external(sd card) storage values such as space used and space available? I cant seem to find an api anywhere?
View 4 Replies View RelatedIn Android, capturing date from datepicker and storing as string in sqlite. Sorting by date doesn't work because they're strings (unless I'm doing it wrong.I've googled this issue for ~5 days, and it looks like there should be a way to capture the date from the date picker, convert it to a Long, store it in sqlite as a Long, select and sort on the Long date value, then convert the Long back to a "mm/dd/yyyy" string for display. I've tried various combinations of parse statements, Date, FormatDate, etc. with no luck at all.On activity start, get today's date and display it in button which calls the datepicker.Capture new date from datepicker (if one is entered), save it as a long to sqlite.On opening an activity showing a listview of records, select from sqlite with orderby on date (Long), convert Long to "mm/dd/yyyy" string for display in ListView.
View 3 Replies View RelatedI have an Android library project that makes calls to PreferencesManager.getDefaultSharedPreferences.
I have 2 version of my app, paid/free, and they are not able to access the preferences stored by the library code.
Can someone tell me the right way to store values in SharedPreferences in library code and have the values available to projects that include the library?
I have an activity with 50 buttons. Want to avoid writing 50 switch cases for onClick listener events. Is their a way to map the buttons with its respective loading of UI in XML format and avoid writing Java code.
View 19 Replies View RelatedI need to create dynamic UI. How can I add margin to the left side of the button.
newPost =new Button(BlogsList.this); newPost.setText("Add Entry To Blog"); newPost.setPadding(10, 10, 10, 10); newPost.setLayoutParams(new LayoutParams (LayoutParams.WRAP_CONTENT,LayoutParams.WRAP_CONTENT)); newPost.setOnClickListener(new NewPost(b.getName (),b.getBlogId())); l22.addView(newPost);
I need to de-serialise a file into an object of a given type. Basically the method will do this:
FileInputStream fis = new FileInputStream(filename);
ObjectInputStream in = new ObjectInputStream(fis);
MyClass newObject = (MyClass)in.readObject();
in.close();
return newObject;
I would like this method to be generic, therefore I can tell it what type I want to in.readObject() to cast its output into, and return it. Hope this makes sense...then again, I probably didn't understand generics properly and this is not actually possible, or advisable.